1.Store 2 numbers in two variables and swap or exchange them by using 3rd variable
class swap1
{
public static void main(String args[])
{
int a=10,b=20,t;
System.out.println(“Before swap: a = ” + a + “, b = ” + b);
t = a;
a = b;
b = t;
System.out.println(“After swap: a = ” + a + “, b = ” + b);
}
}
OR
Store 2 numbers in two variables and swap or exchange them without using 3rd variable
class swap1
{
public static void main(String args[])
{
int a=10,b=20;
System.out.println(“Before swap: a = ” + a + “, b = ” + b);
a= a+b;
b =a-b;
a=a-b;
System.out.println(“After swap: a = ” + a + “, b = ” + b);
}
}
Output:
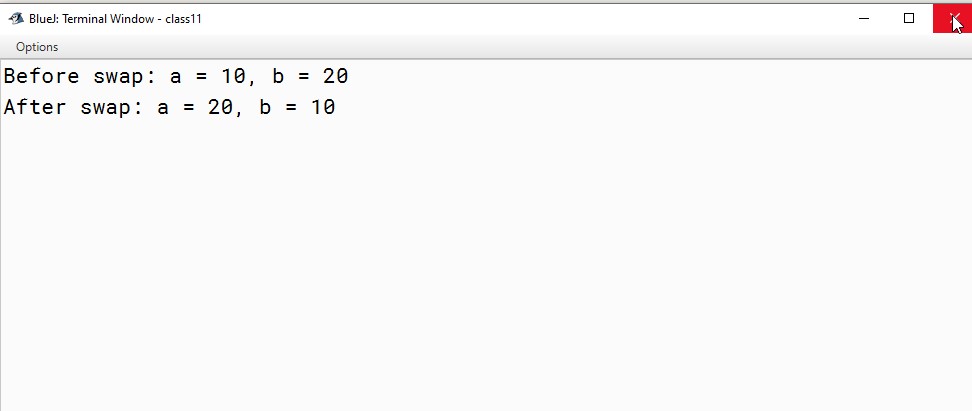
2.Store u,a,t and calculate v and s v=u+at s=ut+1/2 at2 . Print the value of v and s.
class velocity
{
public static void main(String args[])
{
double u=5.5,a=5.6,t=4.5;
double v = u + a * t; // Final velocity
double s = u * t + 0.5 * a * t * t; // Displacement
System.out.println(“Final Velocity (v): ” + v);
System.out.println(“Displacement (s): ” + s);
}
}
Output:
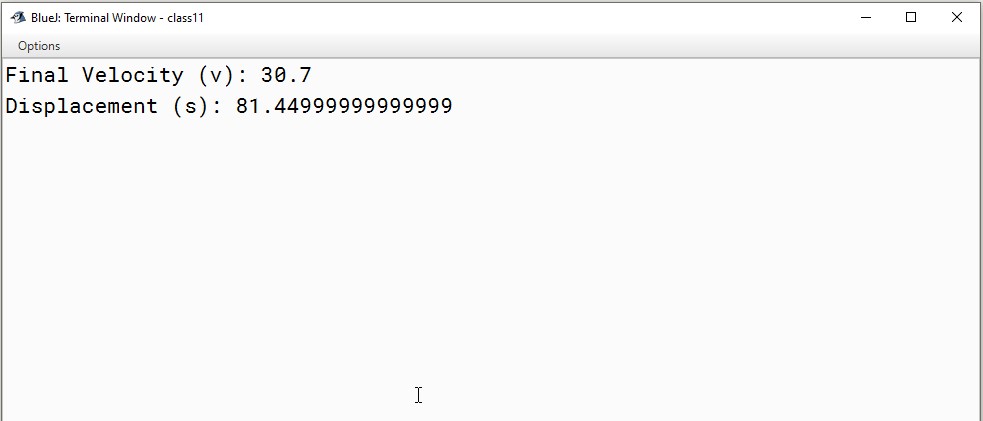
3.Store the values of p,r,t . calculate si and amount and display after calculation.
public class Si {
public static void main(String[] args) {
double p = 1000;
double r = 5;
double t = 2;
double si = (p * r * t) / 100;
double totalAmount = p + si;
System.out.println(“Principal Amount : ” + p);
System.out.println(“Rate of Interest : ” + r + “%”);
System.out.println(“Time Period : ” + t + ” years”);
System.out.println(“Simple Interest : ” + si);
System.out.println(“Total Amount: ” + totalAmount);
}
}
Output:
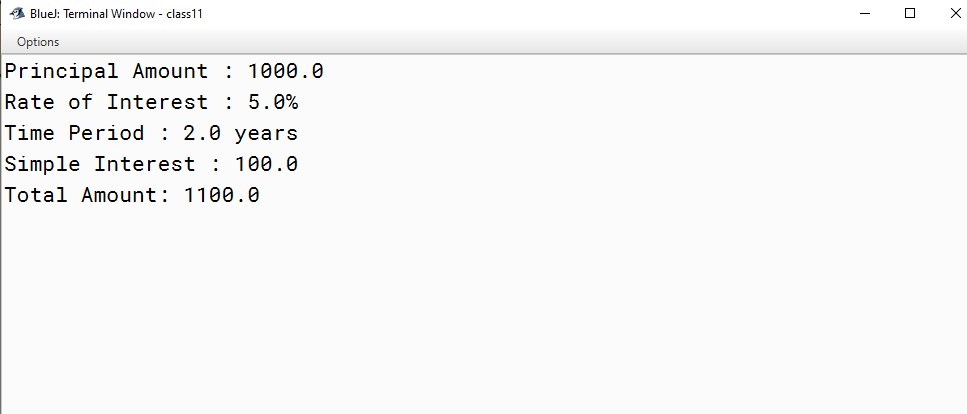
4.Rectangle Area and Perimeter
public class Rectangle {
public static void main(String[] args) {
double length = 10;
double breadth = 5;
double area = length * breadth;
double perimeter = 2 * (length + breadth);
System.out.println(“Length of the Rectangle: ” + length);
System.out.println(“Breadth of the Rectangle: ” + breadth);
System.out.println(“Area: ” + area);
System.out.println(“Perimeter: ” + perimeter);
}
}
Output:
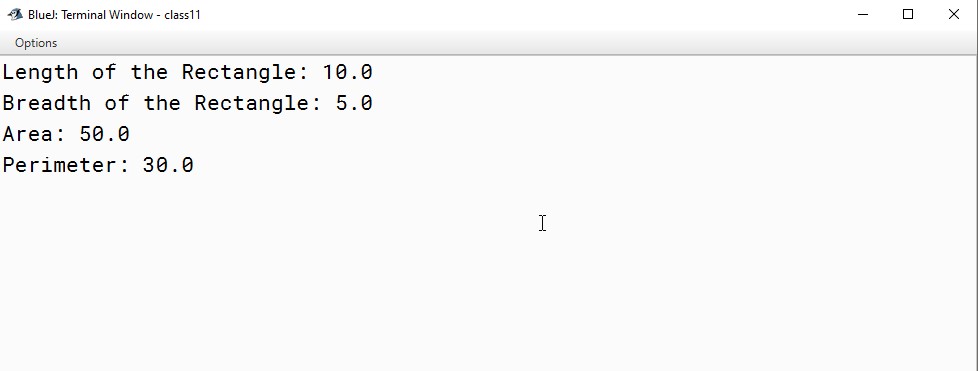
5.Check the greater number among 3 numbers. Include equality test also.
class GreatestNumber {
public static void main(int a,int b,int c) {
if (a > b && a > c)
{
System.out.println(“The greatest number is: ” + a);
}
else if(b>a && b>c)
{
System.out.println(“The greatest number is: ” + b);
}
else if(c>a && c>b)
{
System.out.println(“The greatest number is: ” + c);
}
else if(a==b && b==c)
{
System.out.println(“All are equal”);
}
else{
System.out.println(“unknown condition”);
}
}
}
Output:
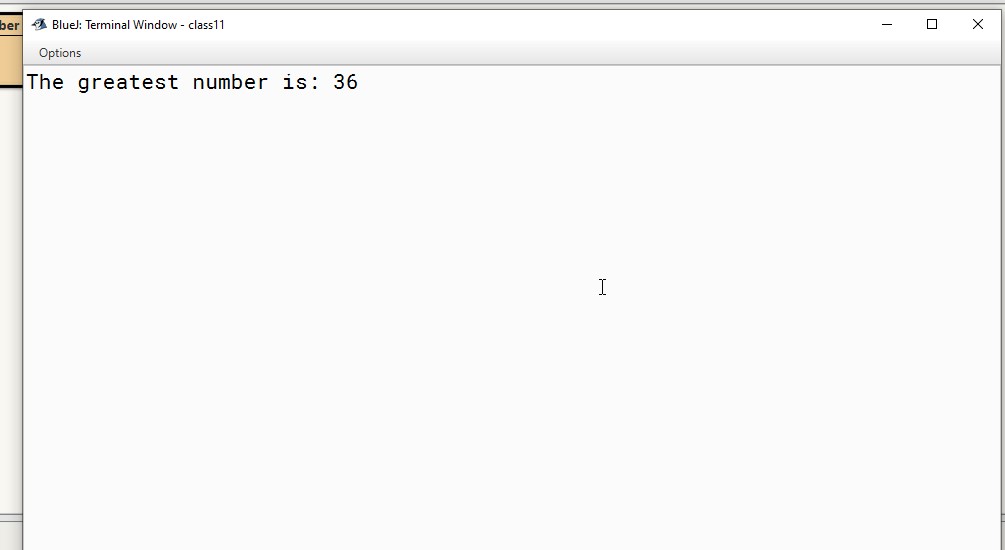
6. No of item 15, price of each as RS 350/-. Give a discount of 5% and add a tax of 3.5% after the discount. Generate and print a bill.
class BillGenerator {
public static void main(String[] args) {
int num= 15;
double pri= 350;
double rate= 5.0;
double tax = 3.5;
double tc = num * pri;
double dis = tc * (rate / 100);
double dispc = tc- dis;
double taxval = dispc * (tax / 100);
double finalBill = dispc + tax;
System.out.println(“Final Bill: ” + finalBill);
}
}
Output:
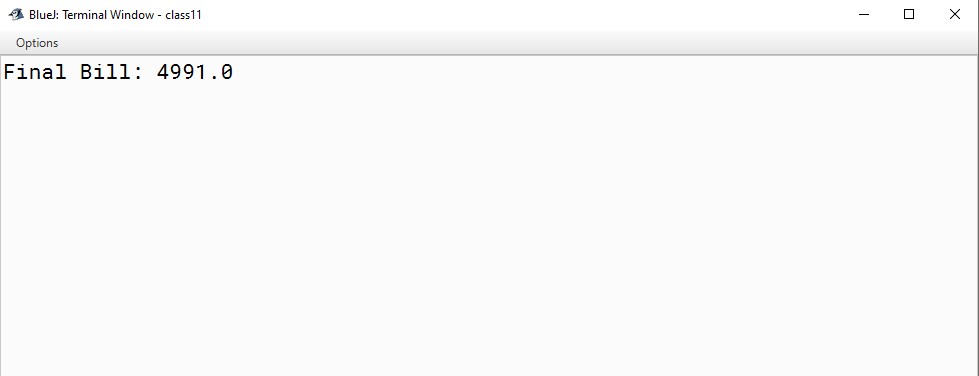
7. Accept 3 sides of a triangle. Check whether the formation is possible or not. If possible then check and print equilateral, isosceles, and scalene triangles.
class TriangleType {
public static void main(int a,int b,int c) {
if (a + b > c && a + c > b && b + c > a) {
System.out.println(“Triangle is valid.”);
if (a == b && b == c) {
System.out.println(“The triangle is Equilateral.”);
} else if (a == b || b == c || a == c) {
System.out.println(“The triangle is Isosceles.”);
} else if (a!=b && b!=c && c!=a)
{
System.out.println(“The triangle is Scalene.”);
}
}
else {
System.out.println(“Triangle is not valid.”);
}
}
}
Output:
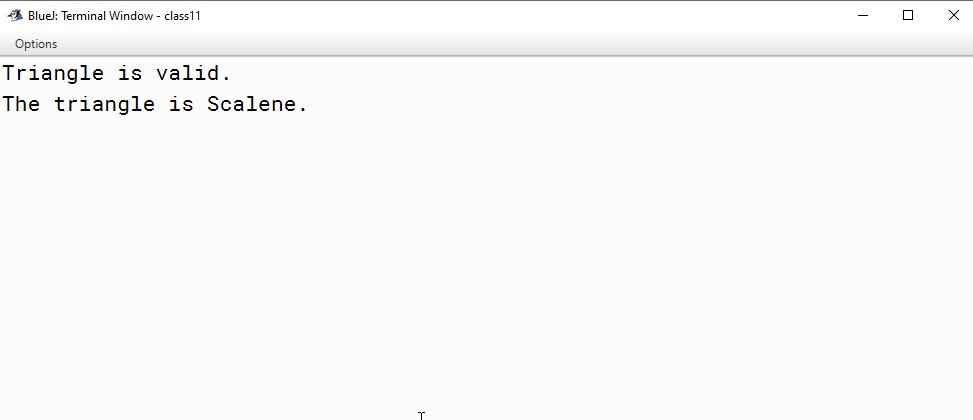
8.Store the price of an item and given discount :
PRICE | DISCOUNT |
>=10000 | 7.5% |
>=5000 AND <10000 | 5.5% |
<5000 | 3.5% |
Add 2.5% tax after discount.
class PriceCalculator {
public static void main(String[] args) {
double price = 12000;
double discount = 0;
double taxRate = 2.5;
if (price >= 10000) {
discount = 7.5;
} else if (price >= 5000) {
discount = 5.5;
} else {
discount = 3.5;
}
double discountAmount = (price * discount) / 100;
double priceAfterDiscount = price – discountAmount;
double taxAmount = (priceAfterDiscount * taxRate) / 100;
double finalPrice = priceAfterDiscount + taxAmount;
System.out.println(“Original Price: ” + price);
System.out.println(“Discount: ” + discount + “%”);
System.out.println(“Price After Discount: ” + priceAfterDiscount);
System.out.println(“Tax: ” + taxRate + “%”);
System.out.println(“Final Price After Tax: ” + finalPrice);
}
}
Output:
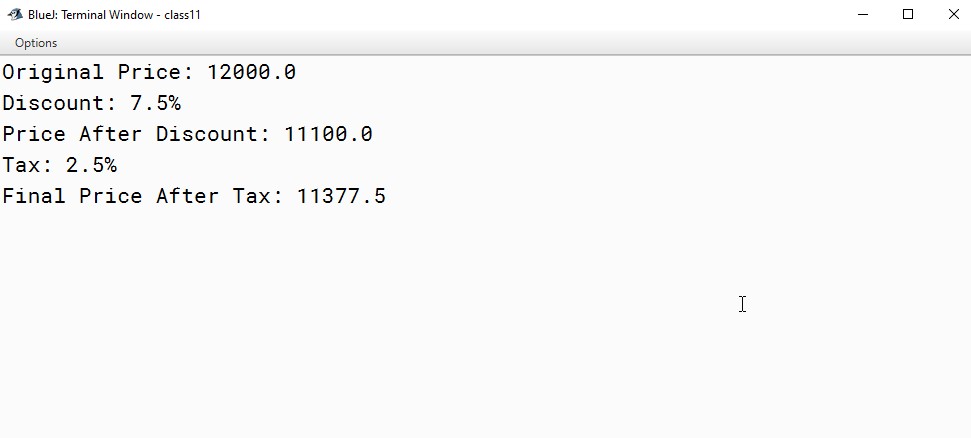
9. Accept a year and check if it is a leap year or not.
class Leapyear {
public static void main(int year)
{
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
System.out.println(year + ” is a leap year.”);
} else {
System.out.println(year + ” is not a leap year.”);
}
}
}
Output:
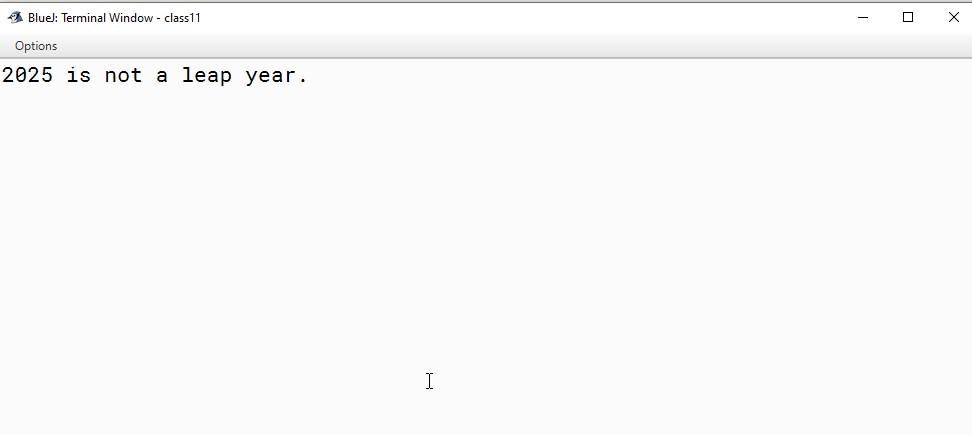
10.Write a Java program to calculate the electricity bill based on the following slabs and add a 20% surcharge to the total bill.
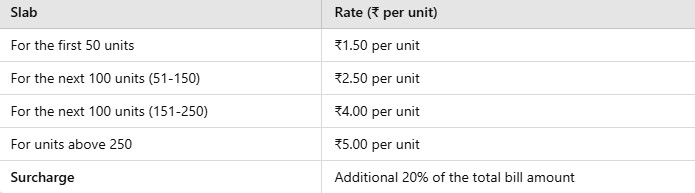
- Predefine the number of units consumed.
- Calculate and display:
- The bill amount before adding the surcharge.
- The final bill amount after adding the surcharge.
class ElectricityBill {
public static void main(String[] args) {
double units = 250;
double billAmount = 0;
if (units <= 50) {
billAmount = units * 1.50;
} else if (units <= 150) {
billAmount = (50 * 1.50) + ((units – 50) * 2.50);
} else if (units <= 250) {
billAmount = (50 * 1.50) + (100 * 2.50) + ((units – 150) * 4.00);
} else {
billAmount = (50 * 1.50) + (100 * 2.50) + (100 * 4.00) + ((units – 250) * 5.00);
}
double finalBill = billAmount + (billAmount * 0.20); // Adding 20% surcharge
System.out.println(“Units Consumed: ” + units);
System.out.println(“Bill Amount: ” + billAmount);
System.out.println(“Final Bill Amount : ” + finalBill);
}
}
Output:
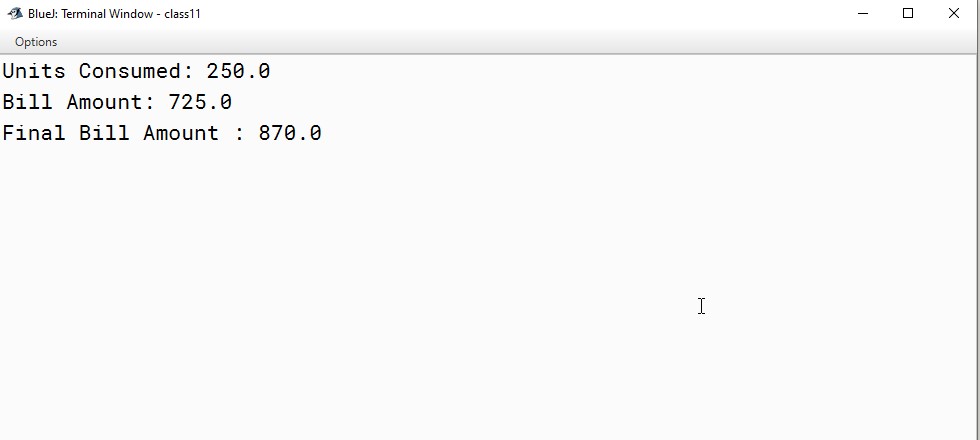
sir nice website
Thanks